Hello,
First,....iam another totally new at the DIY Teensy/Arduino programming world.
My project is a Solar Hot Water Control.
Harware.....
Teensy ++ 2.0.
Home made Tennsy ++ 2.0 terminal board.
Windows XP PC.
Arduino Ver. 1.0.6/Teensyduino Ver. 1.2 software.
Keyes/funduino Relay Board Ver. 8R1A
(2) 10K Thermistor Sensors.
Iam trying to control one relay/water pump with 2 sensors. Example: HotWaterTank sensor says water is cold, turn on relay/water pump, but Collector Tank sensor says no, because temperature is not hot enough in Collector Tank. When Collector Tank gets hot enough, then relay/water pump will be allowed to turn on if Hot Water Tank sensor is wanting pump on. Sorry for "dummy" way of explaining it.
Dont know how to explain it in C language...obviously. 
The below program is what i have so far. (Not working correctly.) It is what i originally put together to control 2 relays with 2 sensors. The below is an attempt to control 1 relay with 2 sensors.
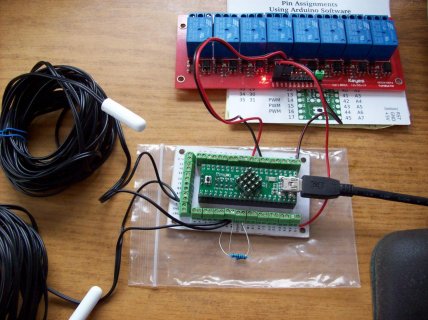
I came up with a way to control pump by using 2 relays, but would rather use only 1 relay if its possible.
Thanks in advance for any info,
Troy
First,....iam another totally new at the DIY Teensy/Arduino programming world.
My project is a Solar Hot Water Control.
Harware.....
Teensy ++ 2.0.
Home made Tennsy ++ 2.0 terminal board.
Windows XP PC.
Arduino Ver. 1.0.6/Teensyduino Ver. 1.2 software.
Keyes/funduino Relay Board Ver. 8R1A
(2) 10K Thermistor Sensors.
Iam trying to control one relay/water pump with 2 sensors. Example: HotWaterTank sensor says water is cold, turn on relay/water pump, but Collector Tank sensor says no, because temperature is not hot enough in Collector Tank. When Collector Tank gets hot enough, then relay/water pump will be allowed to turn on if Hot Water Tank sensor is wanting pump on. Sorry for "dummy" way of explaining it.
The below program is what i have so far. (Not working correctly.) It is what i originally put together to control 2 relays with 2 sensors. The below is an attempt to control 1 relay with 2 sensors.
Code:
void setup()
{
Serial.begin(38400);
pinMode(PIN_D7, OUTPUT);
pinMode(PIN_D5, OUTPUT);
}
float code;
float celsius;
float fahrenheit;
void loop()
{
code = analogRead(0);
celsius = 25 + (code - 512) / 11.00;
fahrenheit = celsius * 1.8 + 32;
Serial.print("HotWaterTank: ");
Serial.print(celsius);
Serial.print(" Celsius, ");
Serial.print(fahrenheit);
Serial.println(" Fahrenheit");
if (celsius <27)
{
digitalWrite(PIN_D7, HIGH); // Relay1 ON
}
else
{
digitalWrite(PIN_D7, LOW); // Relay1 OFF
}
code = analogRead(1);
celsius = 25 + (code - 512) / 11.00;
fahrenheit = celsius * 1.8 + 32;
Serial.print("CollectorTank: ");
Serial.print(celsius);
Serial.print(" Celsius, ");
Serial.print(fahrenheit);
Serial.println(" Fahrenheit");
if (celsius <25)
{
digitalWrite(PIN_D7, LOW); // Relay1 OFF
}
else
{
digitalWrite(PIN_D7, HIGH); // Relay1 ON
}
delay(5000);
}
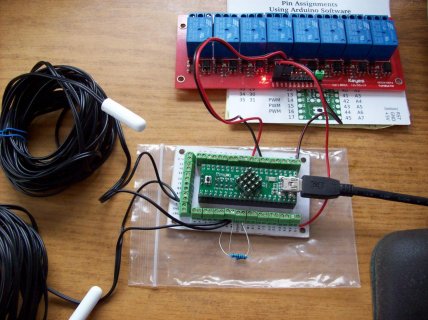
I came up with a way to control pump by using 2 relays, but would rather use only 1 relay if its possible.
Thanks in advance for any info,
Troy
Last edited: