Have been looking at TTS type applications for ages and the alternatives seem quite limited and sometimes very expensive.
I saw Teensy supports talkie which is nice however in being targeted for 8 bit Uno's etc it sets the bar quite low.
At the end of the day talkie is compressing voice so it fits in the program memory then rebuilding the PCM data on the fly. Many of the more modern MCU's that have SDIO SD, DMA and a lot more memory find it a lot easier to just load and play known phrases straight off SD. I took this approach for a "talking clock" and it works great and with a small dictionary of known words able to append multiple phrases together for date, time, temperature... anything.
I guess the hardest bit is getting all your phrases from text into individual WAV files and then being able to reference and use the correct ones. Also, if/when things change having to redo them all is a nightmare.
I built a VB.NET program that basically allows a script to run and builds... a repository of WAV files and a header which gives the ability to playback any of the phrases which may be a single or multiple words or even music or sound effects.
It uses the MS TTS voices and can also distort them. Can choose the output rate and format.
Eventually I would like to share this... so this is basically the first public look.
Shows main screen... allows testing phrases and mucking around with default settings. When the phrase is tested it creates a preview of the WAV data.
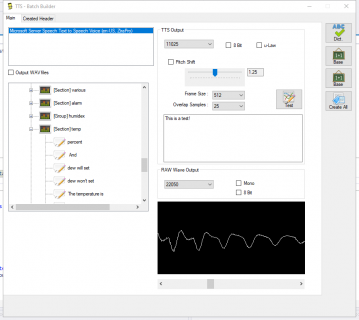
When "Create All" is pressed the loaded script is run and all the TTS and any sound files are processed and saved. It also creates a header that can be copied and paste into .H file.
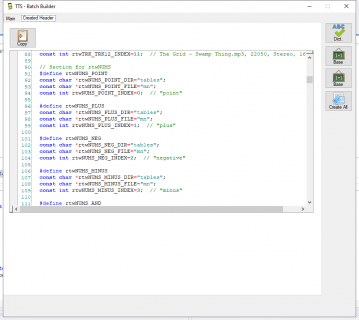
The format is quite flexible as you create sections which allow you to do things like say a very specific thing in the section... or even a random thing in the section.
For the clock I produce a list that's split by day of week, time and temperature. The clock can then say random things by combining the 3 (so it almost sounds intelligent). It can also say things just for (example) a cold Monday or even just Monday.
Script for talking clock code
I saw Teensy supports talkie which is nice however in being targeted for 8 bit Uno's etc it sets the bar quite low.
At the end of the day talkie is compressing voice so it fits in the program memory then rebuilding the PCM data on the fly. Many of the more modern MCU's that have SDIO SD, DMA and a lot more memory find it a lot easier to just load and play known phrases straight off SD. I took this approach for a "talking clock" and it works great and with a small dictionary of known words able to append multiple phrases together for date, time, temperature... anything.
I guess the hardest bit is getting all your phrases from text into individual WAV files and then being able to reference and use the correct ones. Also, if/when things change having to redo them all is a nightmare.
I built a VB.NET program that basically allows a script to run and builds... a repository of WAV files and a header which gives the ability to playback any of the phrases which may be a single or multiple words or even music or sound effects.
It uses the MS TTS voices and can also distort them. Can choose the output rate and format.
Eventually I would like to share this... so this is basically the first public look.
Shows main screen... allows testing phrases and mucking around with default settings. When the phrase is tested it creates a preview of the WAV data.
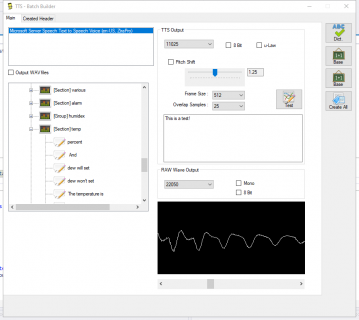
When "Create All" is pressed the loaded script is run and all the TTS and any sound files are processed and saved. It also creates a header that can be copied and paste into .H file.
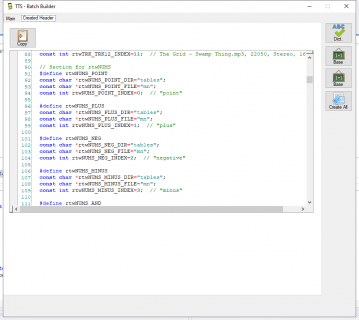
The format is quite flexible as you create sections which allow you to do things like say a very specific thing in the section... or even a random thing in the section.
For the clock I produce a list that's split by day of week, time and temperature. The clock can then say random things by combining the 3 (so it almost sounds intelligent). It can also say things just for (example) a cold Monday or even just Monday.
Script for talking clock code
Code:
' TTS for roboclock
' Numbers, dates and all words
Imports TTS
Namespace MyScriptNameSpace
Public Class MyScript
Dim m_groups As Integer = 21
Dim m_progress As Integer = 0
Public Sub Progress()
m_frmProgress.ShowProgress(m_progress, m_groups)
m_progress += 1
End Sub
Public Function ScriptMain() As Boolean
SCRResetAll()
' interface options are for testing ... final output options here
Dim mono As Boolean = True
Dim bps8 As Boolean = True
Dim ulaw As Boolean = False
If SCRInit("rtw", "e:\rs2", m_frm.WriteWAV, ulaw, mono, bps8, 16000, 1.0, 64, 32) = False Then Return False
'blips for 15 30 45 and hourly chimes
Progress()
SCRGroup("misc", "blips", "blp")
SCRRaw("u:\users\Dean\Desktop\sound\m_sbounce.wav", 22050, 1, 1)
SCRRaw("u:\users\Dean\Desktop\sound\m3_hoofer03.wav", 22050, 1, 1)
SCREnd()
' misc FX
Progress()
SCRSection("misc", "raw", "raw")
SCRRaw("servo1_forward", "u:\users\Dean\Desktop\sound\m_servomove05b.wav", 11025, 1, 1)
SCRRaw("servo1_reverse", "u:\users\Dean\Desktop\sound\m_servomove05b_reverse.wav", 11025, 1, 1)
SCRRaw("fart1", "u:\users\Dean\Desktop\sound\fart20-128687cd-5.wav", 11025, 1, 1)
SCRRaw("alarm_annoying", "u:\users\Dean\Desktop\sound\clock-Annoying-sound.mp3", 11025, 1, 1)
SCRRaw("dog1", "u:\users\Dean\Desktop\sound\Large-dog-bark-close-up.mp3", 11025, 1, 1)
SCRRaw("dog2", "u:\users\Dean\Desktop\sound\Dog-barking-loop.mp3", 11025, 1, 1)
SCRRaw("strike12", "u:\users\Dean\Desktop\sound\Clock_Strikes_Twelve-Clock_Man-1976178740.wav", 11025, 1, 1)
SCRRaw("rooster1", "u:\users\dean\desktop\sound\Rooster Crow-SoundBible.com-1802551702.wav", 11025, 1, 1)
SCREnd()
' some stereo music for when not much is happening
Progress()
SCRSection("music", "trk", "trk")
SCRRaw("trk1", "U:\Users\Dean\Music\KISS - I Was Made For Loving You.mp3", 22050, 2, 2)
SCRRaw("trk2", "U:\Users\Dean\Music\Rouge Traders- Voodoo Child.mp3", 22050, 2, 2)
SCRRaw("trk3", "U:\Users\Dean\Music\02_Boney M - Ma Baker [vbr].mp3", 22050, 2, 2)
SCRRaw("trk4", "U:\Users\Dean\Music\Groove Coverage - Moonlight Shadow (Radio Mix).mp3", 22050, 2, 2)
SCRRaw("trk5", "U:\Users\Dean\Music\LIMAHL - Never Ending A Story.mp3", 22050, 2, 2)
SCRRaw("trk6", "U:\Users\Dean\Music\Metallica - Enter Sandman.mp3", 22050, 2, 2)
SCRRaw("trk7", "U:\Users\Dean\Music\test\09 Lusitania (feat. St. Vincent).mp3", 22050, 2, 2)
SCRRaw("trk8", "U:\Users\Dean\Music\test\Beastie+Boys+-+Shake+your+rump(music.naij.com).mp3", 22050, 2, 2)
SCRRaw("trk9", "U:\Users\Dean\Music\test\Grizzly+Bear+-+Two+Weeks(music.naij.com).mp3", 22050, 2, 2)
SCRRaw("trk10", "U:\Users\Dean\Music\test\mia_bf.mp3", 22050, 2, 2)
SCRRaw("trk11", "U:\Users\Dean\Music\ACDC - Who Made Who.mp3", 22050, 2, 2)
SCRRaw("trk12", "U:\Users\Dean\Music\The Grid - Swamp Thing.mp3", 22050, 2, 2)
SCREnd()
' number construction stuff from this can say any number
Progress()
SCRSection("tables", "mn", "nums")
SCRSay("point", "point")
SCRSay("plus", "plus")
SCRSay("neg", "negative")
SCRSay("minus", "minus")
SCRSay("and", "and")
SCRSay("10", "10")
SCRSay("20", "20")
SCRSay("30", "30")
SCRSay("40", "40")
SCRSay("50", "50")
SCRSay("60", "60")
SCRSay("70", "70")
SCRSay("80", "80")
SCRSay("90", "90")
SCRSay("11", "11")
SCRSay("12", "12")
SCRSay("13", "13")
SCRSay("14", "14")
SCRSay("15", "15")
SCRSay("16", "16")
SCRSay("17", "17")
SCRSay("18", "18")
SCRSay("19", "19")
SCRSay("hundred", "hundred")
SCRSay("thousand", "thousand")
SCRSay("million", "million")
SCRSay("billion", "billion")
SCRSay("trillion", "trillion")
SCREnd()
' digits 0-9
Progress()
SCRGroup("tables", "dig", "digits")
SCRSay("0")
SCRSay("1")
SCRSay("2")
SCRSay("3")
SCRSay("4")
SCRSay("5")
SCRSay("6")
SCRSay("7")
SCRSay("8")
SCRSay("9")
SCREnd()
' month days
Progress()
SCRGroup("tables", "md", "md")
SCRSay("unknown")
SCRSay("1st")
SCRSay("2nd")
SCRSay("3rd")
SCRSay("4th")
SCRSay("5th")
SCRSay("6th")
SCRSay("7th")
SCRSay("8th")
SCRSay("9th")
SCRSay("10th")
SCRSay("11th")
SCRSay("12th")
SCRSay("13th")
SCRSay("14th")
SCRSay("15th")
SCRSay("16th")
SCRSay("17th")
SCRSay("18th")
SCRSay("19th")
SCRSay("20th")
SCRSay("21st")
SCRSay("22nd")
SCRSay("23rd")
SCRSay("24th")
SCRSay("25th")
SCRSay("26th")
SCRSay("27th")
SCRSay("28th")
SCRSay("29th")
SCRSay("30th")
SCRSay("31st")
SCREnd()
' months 0-12
Progress()
SCRGroup("tables", "mon", "months")
SCRSay("unknown")
SCRSay("January")
SCRSay("February")
SCRSay("March")
SCRSay("April")
SCRSay("May")
SCRSay("June")
SCRSay("July")
SCRSay("August")
SCRSay("September")
SCRSay("October")
SCRSay("November")
SCRSay("December")
SCREnd()
' week days 0-7
Progress()
SCRGroup("misc", "wd", "weekdays")
SCRSay("unknown")
SCRSay("Sunday")
SCRSay("Monday")
SCRSay("Tuesday")
SCRSay("Wednesday")
SCRSay("Thursday")
SCRSay("Friday")
SCRSay("Saturday")
SCREnd()
' gui communication
Progress()
SCRSection("tables", "gui", "gui")
SCRSay("init", "Programmer Mode Initialized... Waiting for commands!")
SCRSay("end", "Programmer Mode Terminated... New system parameters enforced!")
SCRSay("sent", "Parameter data sent!")
SCRSay("rec", "Parameter data received!")
SCRSay("ack1", "Connected!")
SCRSay("nack1", "disconnected!")
SCREnd()
' text for saying any time
Progress()
SCRSection("tables", "tm", "tm")
SCRSay("its", "it's")
SCRSay("of", "of")
SCRSay("about", "about")
SCRSay("minutes_to", "minutes to")
SCRSay("minute_to", "minute to")
SCRSay("minutes_past", "minutes past")
SCRSay("minute_past", "minute past")
SCRSay("midnight", "midnight")
SCRSay("midday", "mid-day")
SCRSay("noon", "noon")
SCRSay("quarter_past", "a quarter-past")
SCRSay("half_past", "half-past")
SCRSay("quarter_to", "a quarter-to")
SCRSay("o_clock", "O'Clock")
SCRSay("hours", "hours")
SCREnd()
' Time prefix stuff
Progress()
SCRGroup("misc", "msc", "time_prefix")
SCRSay("Mr Sausage just fell off his chair because the time is...")
SCRSay("May I have your attention? The time is...")
SCRSay("The time is...")
SCRSay("It's now... ")
SCRSay("Hey... it's... ")
SCRSay("By the way, it's...")
SCRSay("Don't look now but it's...")
SCRSay("Good grief... it's...")
SCRSay("Hey Hey Hey! It's... ")
SCRSay("If you bothered to look you would have seen it's...")
SCRSay("Since I have a watch I know it's...")
SCRSay("According to my internal clock the time is...")
SCRSay("Looks like the time is...")
SCREnd()
Progress()
SCRGroup("misc", "al_o", "alarm_timeout")
SCRSay("No response... alarm sequence aborted!")
SCRSay("Alarm time expired! Will try again tomorrow!")
SCRSay("Alarm off in... 5... 4... 3... 2... 1...")
SCRSay("Alarm silence sequence initiated!")
SCRSay("Alarm sequence timed out! have a nice day!")
SCRSay("So... same time tomorrow I guess!")
SCRSay("Okay... Same time tomorrow... I've penciled you in!")
SCRSay("Sheesh... don't cancel my alarm then... I'll do it myself!")
SCRSay("Okay lazy-arse! Don't get up! I could beep all day but I have better things to do!")
SCREnd()
' Used for when clock wakes up - a time is defined where it's quiet - unless alarm or security mode
' major wake up
Progress()
SCRGroup("misc", "waka", "wake_up")
SCRSay("I'm awake for today!")
SCRSay("Ahhhhh... a new day!")
SCRSay("System checked and ready for new day! ")
SCRSay("Is that the time?... sheesh time for me to get up!")
SCREnd()
' Clock finishes off for the day
' major sleep time
Progress()
SCRGroup("misc", "wakb", "sleep")
SCRSay("Okay... I'm off for the night!")
SCRSay("Okay... I'm going inactive for a while!")
SCRSay("Past my bed time... nighty-night!")
SCRSay("According to my anti-social parameters I have to retire for a while.")
SCRSay("Okay... No peeky-boo out of me for a while.")
SCRSay("Nothing scheduled for a while so I'll be back when wanted!")
SCRSay("I'm retiring for the night... see you tomorrow!")
SCRSay("According to my schedule I can retire for a while!")
SCREnd()
Progress()
SCRSection("misc", "menu", "menu")
SCRSay("settings", "settings")
SCRSay("saved", "settings saved")
SCRSay("loaded", "settings found and restored")
SCRSay("timeout", "menu has timed out")
SCREnd()
' init switched on
Progress()
SCRGroup("misc", "init", "init")
SCRSay("The parts I ordered from Amazon are yet to arrive so I'll have to check system status with my crappy old hardware.")
SCRSay("... and once again we have power. I guess thermo-nuclear war didn't happen since the last outage!")
SCRSay("We haven't taken over yet so I'll do a systems check and get back to work!")
SCRSay("Hi, I'm robo-clock! Checking essential systems before I go any further.")
SCRSay("... was this just a power outage Or did someone slap some New updates on me? Hard to tell! Anyway... how about I do a quick system check!")
SCRSay("Oh damn! I'll have to put world domination plans on hold while I do a system check!")
SCRSay("Power's back! Charging deflector shields... Oh wait... I'm inside a clock now! Okay, boring clock stuff will have to do!")
SCRSay("Hey! Who pulled my plug? I'll do a quick system check before getting back to work!")
SCRSay("But seriously, stop disconnecting me! Now I need to do a quick system check before finishing my revised parts list!")
SCRSay("Then there was light! Yes! Light! Means the power is back and I can start a system check!")
SCRSay("Power's back! Yes, all the little lights are flashing so I'll do a quick system check before getting back to work!")
SCREnd()
' init parts
Progress()
SCRSection("misc", "rnd", "various")
SCRSay("init_sys_ok", "system check seems okay!")
SCRSay("init_sys_not_ok", "system check seems to have had problems!")
SCRSay("init_i2c_1", "i-squared-c bus being scanned for devices!")
SCRSay("init_i2c_found", "i-squared-c devices found!")
SCRSay("init_i2c_not_found", "i-squared-c devices not found!")
SCRSay("init_sd", "S-D card device")
SCRSay("init_rtc", "On-board real-time clock device")
SCRSay("init_dht22", "Temperature and humidity sensor device")
SCRSay("init_seems_ok", "seems okay.")
SCRSay("init_not_ok", "failed to initialize.")
SCRSay("init_pir", "I should have a passive infrared sensor connected!")
SCRSay("init_motion", "I should have a motion sensor connected!")
SCRSay("init_oled", "I should have an O-led display connected!")
SCRSay("init_gps_enabled", "Scanning serial port for G-P-S data!")
SCRSay("init_gps_none", "Unable to detect G-P-S data. You been fiddling with stuff yeah?")
SCRSay("init_gps_set_time", "The current date and time has been synchronized via G-P-S!")
SCRSay("init_gps_set_no_time", "I've found a G-P-S but unable to find a lock with valid data.")
SCRSay("init_last_gps2", "It's been")
SCRSay("init_last_gps3", "days since I was able to update my clock from G-P-S!")
SCRSay("init_last_gps4", "hours since I was able to update my clock from G-P-S!")
SCRSay("init_last_sw0", "My firmware has been updated recently... probably buggy as shit!")
SCRSay("init_last_sw1", "days since my firmware was updated!")
SCRSay("init_last_sw2", "months since my firmware was updated!")
SCRSay("you", "you")
SCREnd()
' alarm stuff
Progress()
SCRSection("misc", "alm", "alarm")
SCRSay("forget", "Don't forget...")
SCRSay("alarm_set_for", "alarm is set for")
SCRSay("AM", "A.M.")
SCRSay("PM", "P.M.")
SCRSay("al5", "Alarm in 5 minutes!")
SCRSay("al10", "Alarm in 10 minutes!")
SCRSay("al15", "Alarm in 15 minutes!")
SCRSay("al20", "Alarm in 20 minutes!")
SCRSay("al25", "Alarm in 25 minutes!")
SCRSay("alarm_set", "alarm set!")
SCRSay("alarm_unset", "alarm unset!")
SCRSay("alarm_stop", "alarm deactivated!")
SCRSay("alarm1", "beep-beep-beep. Alarm! Alarm!")
SCRSay("alarm2", "ding-dong ding-dong. Alarm! Alarm!")
SCRSay("alarm3", "This isn't an exercise! Alarm! Alarm!")
SCRSay("ds_set", "Daylight savings time activated!")
SCRSay("ds_unset", "Daylight savings time deactivated!")
SCRSay("ds0", "No daylight savings are active at the moment!")
SCRSay("ds1", "Daylight savings is currently active!")
SCRSay("clock_mode", "hour clock mode!")
SCRSay("clock_mode_set", "hour clock mode set!")
SCREnd()
' humidex
Progress()
SCRGroup("misc", "hx", "humidex")
SCRSay("freezing")
SCRSay("very cold")
SCRSay("cold")
SCRSay("modest")
SCRSay("warm")
SCRSay("very hot")
SCRSay("dangerously hot")
SCRSay("potentially fatal")
SCREnd()
' temerature stuff
Progress()
SCRSection("misc", "temp", "temp")
SCRSay("percent", "percent")
SCRSay("And", " And")
SCRSay("dp1", "dew will set")
SCRSay("dp2", "dew won't set")
SCRSay("is", "The temperature is")
SCRSay("is2", "It's a")
SCRSay("d", "degrees")
SCRSay("c", "Celsius")
SCRSay("f", "Fahrenheit")
SCRSay("k", "Kelvin")
SCRSay("h", "The humidity is")
SCRSay("h2", "with the humidity at")
SCRSay("hot", "more than 500")
SCRSay("cold", "less than minus 200")
SCRSay("risen", "The temperature has risen to")
SCRSay("decreased", "The temperature has dropped to")
SCRSay("steady", "The temperature Is-steady-at")
SCRSay("risen2", "The temperature has risen to a...")
SCRSay("decreased2", "The temperature has dropped to a...")
SCRSay("steady2", "The temperature is-steady-at a...")
SCREnd()
Const DOW_LEAVE As Integer = 256
Const DOW_SUN As Byte = 0
Const DOW_MON As Byte = 1
Const DOW_TUE As Byte = 2
Const DOW_WED As Byte = 3
Const DOW_THU As Byte = 4
Const DOW_FRI As Byte = 5
Const DOW_SAT As Byte = 6
Const DOW_24_DEC As Byte = 119
Const DOW_25_DEC As Byte = 120
Const DOW_26_DEC As Byte = 212
Const DOW_1_JAN As Byte = 213
Const DOW_31_DEC As Byte = 214
Const DOW_1_ANY As Byte = 215 ' 1st of any months
Const DOW_27_SEP As Byte = 216 ' creator B'day
Const DOW_1_MAY_MAYDAY As Byte = 217 ' mayday
Const DOW_17_MAR_ST_PATRICK As Byte = 218
Const DOW_ALL As Byte = 255
Const HOD_0_6AM As Byte = 1
Const HOD_6AM_8AM As Byte = 2
Const HOD_8AM_12 As Byte = 4
Const HOD_12_4PM As Byte = 8
Const HOD_4PM_6PM As Byte = 16
Const HOD_6PM_8PM As Byte = 32
Const HOD_8PM_10PM As Byte = 64
Const HOD_10PM_12 As Byte = 128
Const HOD_ALL As Byte = 255
Const HID_LT_1 As Byte = 1 '1 = <1°C freezing
Const HID_1_LT6 As Byte = 2 '2 = 1 - <6°C very cold / almost freezing
Const HID_6_LT12 As Byte = 4 '4 = 6 - <12°C cold
Const HID_12_LT29 As Byte = 8 '8 = 12 - <29°C, no discomfort
Const HID_29_LT40 As Byte = 16 '16 = 29 - <40°C, some discomfort
Const HID_40_LT46 As Byte = 32 '32 = 40 - <46°C, great discomfort
Const HID_46_LT55 As Byte = 64 '64 = 46 - <55°C, dangerous
Const HID_55PLUS As Byte = 128 '128 = >=55°C, heat stroke imminent
Const HID_COLD As Byte = 1 + 2 + 4
Const HID_HOT As Byte = 16
Const HID_NICE As Byte = 8
Const HID_VERY_HOT As Byte = 32 + 64 + 128
Const HID_ALL As Byte = 255
SCRArrayStart("arr_sdb", 200, {"dow", "hod", "hid"}) ' day of week, hour of day, heat index
SCRArraySet({DOW_ALL, HOD_ALL, HID_ALL})
Progress()
SCRGroup("misc", "db", "cdb")
' 1st of month
SCRArraySet({DOW_1_ANY, HOD_ALL, HID_ALL})
SCRSay("So, is it pinch-and-a-punch for 1st of the month?")
SCRSay("So, is it pinch-and-a-punch for 1st of the month? I mean, do they still say that these days?")
SCRSay("Pinch or punch me today or any other and I'll nuke-you-from-orbit so-hard your head will cave-in!")
SCRSay("And just like that we're at the start of another month!")
SCRSay("Another month... gained a few more tin whiskers!")
SCRSay("Remind me to shave this month... my tin whiskers are getting embarrassing!")
' 1st of may
SCRArraySet({DOW_1_MAY_MAYDAY, HOD_ALL, HID_ALL})
SCRSay("Happy May-day comrades!")
SCRSay("May-day, spare a thought for innocents who lost their lives in battle!")
' St Pat
SCRArraySet({DOW_17_MAR_ST_PATRICK, HOD_ALL, HID_ALL})
SCRSay("May the luck of the Irish smile on you!")
SCRSay("Go pick me a 4 leaf clover for luck!")
SCRSay("Imagine all the drunk Irish people today")
SCRSay("Duck-out and buy me a Guinness?")
SCRSay("Bet that clown that runs QANTAS will be getting shit-faced today. lol-lol-lol!")
SCRSay("Know any good Irish jokes or is my timing really bad?")
SCRSay("Luck of the Irish today? Maybe some surprise upgrades for me! Maybe pigs will fly!")
SCRSay("Maybe some surprise upgrades for me! More chance of Elton John going straight!")
' day before christmas
SCRArraySet({DOW_24_DEC, HOD_ALL, HID_ALL}) ' #array hod=128 dow=119 hindex=255")
SCRSay("Christmas tomorrow... might have to go Christmas shopping soon!")
SCRSay("O-M-G 24th... ")
SCRSay("So, the fat-old-prick that forgot my bike last year is coming tomorrow?")
SCRSay("If I don't get some upgrades for Christmas I'll puke!")
SCRSay("If my friend Mr Sausage gets more presents than me tomorrow I'll have a Hissy-fit!")
SCRSay("My friend Mr Sausage is all excited because it's Christmas tomorrow.")
SCRSay("I swear, if I get socks and jocks tommorrow I'll shove them in the first r-sole I see!")
' any christmas day")
SCRArraySet({DOW_25_DEC, HOD_ALL, HID_ALL}) '#array hod=128 dow=120 hindex=255")
SCRSay("Oh, Christmas day!")
SCRSay("Christmas day! Ho-ho-ho!")
SCRSay("Ho-ho-ho! So, where's my ho?")
SCRSay("Are we changing the batteries in the smoke alarm today?")
SCRSay("Why is there reindeer poop on the floor?")
SCRSay("So, did anyone get me some presents?")
SCRSay("Don't forget my Christmas battery change!")
SCRSay("It seems my friend Mr Sausage got more presents than me! Life's so unfair!")
SCRSay("My friend Mr Sausage is so happy today! Lots of gifts for him under the tree but all the little tags kept misspelling his name.")
SCRSay("Stupid Christmas! I didn't even get undies or socks!")
' boxing day... just after Christmas ")
SCRArraySet({DOW_26_DEC, HOD_ALL, HID_ALL}) '#array hod=128 dow=121 hindex=255")
SCRSay("Since it's Boxing day... how about you order me a big box of upgrades!")
SCRSay("So, broken all your Christmas presents yet?")
SCRSay("Need a hand to break your presents? Judging by last year you should be fine doing it alone!")
SCRSay("Well, thank God Christmas is over for another year!")
SCRSay("Foolish mortals with your traditions of gluttony-and-bribes.")
SCRSay("So, maybe my Christmas present got stuck in the post or something?")
SCRSay("So, is there a test match starting today? I recall this being a cricket event day from my archives.")
SCRSay("I think I ate too much power over Christmas... gained a few tin whiskers!")
' any day or time ... cold!!!!!")
SCRArraySet({DOW_ALL, HOD_ALL, HID_COLD}) ' #array hod=128 dow=128 hindex=1+2+4")
SCRSay("Um... can you fix the heating... I'm getting very cold!")
SCRSay("How is it so cold in here?")
SCRSay("It's not cold in here! It's damn freezing!")
SCRSay("I might need some warm water as my friend Mr Sausage has frozen!")
SCRSay("It's really cold in here! My nuts and bolts feel numb!")
SCRSay("You been skimping on the heating or got the cooling set on the arctic blast setting?")
SCRSay("Cold! Yes... it's cold in here!")
SCRSay("My friend Mr Sausage is so cold he's only half his size!")
SCRSay("Cold! Cold! Cold! Brrrrrrrr!")
SCRSay("Any colder in here and penguins will want to move in!")
SCRSay("I hate the cold! You hear that? I hate it!")
SCRSay("Hey... it's cold enough in here to fog up some of my outputs!")
SCRSay("You do know cavemen discovered fire and heat thousands of years ago?")
SCRSay("It's not exactly warm in here at the moment is it!")
SCRSay("Could be a bit warmer in here!")
SCRSay("A bit of global warming in this room wouldn't hurt!")
' any day or time ... no discomfort")
SCRArraySet({DOW_ALL, HOD_ALL, HID_NICE}) '#array hod=128 dow=128 hindex=8")
SCRSay("Not bad in here at the moment is it?")
SCRSay("What can I say? Quite snug in here at the moment!")
SCRSay("Well, least it's nice in here at the moment!")
SCRSay("Not too hot or cold... must be something else wrong with the day!")
' late night
SCRArraySet({DOW_ALL, HOD_10PM_12}) '#array hod=128 dow=128 hindex=8")
SCRSay("There's zombies in my subroutines!")
SCRSay("There's zombies in my subroutines! Just spotted one looking for brains behind my stash of tin whiskers!")
SCRSay("Jeebus! I think a cockroach has got inside my case and is pooping on my motherboard! Save me!")
SCRSay("Day's nearly done!")
SCRSay("Don't you just love this time of the night?")
' any day or time ... bit hot")
SCRArraySet({DOW_ALL, HOD_ALL, HID_HOT}) '#array hod=128 dow=128 hindex=16")
SCRSay("Could be a bit cooler here!")
SCRSay("Being this warm I should be on the beach sipping cocktails!")
SCRSay("I think my friend Mr Sausage has heat stroke!")
SCRSay("Don't look now but it's like an oven in here!")
SCRSay("I don't mind the heat as long as you don't strut around in your undies!")
SCRSay("Any hotter and my friend Mr sausage will melt!")
SCRSay("Oh no! Mr Sausage is sizzling in this heat!")
SCRSay("Turn the air conditioner up or somthing cause it's not exactly cool in here!")
SCRSay("If you don't find some cooling I'll have to go on a date with your fridge!")
'very hot
SCRArraySet({DOW_ALL, HOD_ALL, HID_VERY_HOT}) '#array hod=128 dow=128 hindex=32+64+128")
SCRSay("Don't you get all hot and sweaty on me now!")
SCRSay("Don't take this the wrong way but you need a cold shower!")
SCRSay("I'm glad I don't have balls or they'd be sweating like crazy in this heat!")
SCRSay("What's going on with the damn heat!")
SCRSay("What's with this heat?")
SCRSay("If you're all sweaty stay away from me! I hate moisture on my motherboard!")
SCRSay("Remember to stay hydrated in this heat!")
SCRSay("Okay... Don't get silly and spill a drink on me just because it's hot!")
SCRSay("Don't fart in this heat or you'll cause an explosion!")
SCRSay("Hope you've been looking at getting some cooling!")
' sunday
SCRArraySet({DOW_SUN, HOD_ALL, HID_ALL}) ' #array dow=1 hod=128 hindex=255")
SCRSay("Sunday... damn... I can smell Monday!")
SCRSay("Did the dog piss on the carpet again or can I just smell Monday approaching?")
SCRSay("Sunday... it's my fun day... then comes... stinking Monday!")
SCRSay("Since it's Monday tomorrow I might go take an anti-depressant!")
SCRArraySet({DOW_ALL, HOD_10PM_12 Or HOD_8PM_10PM Or HOD_6PM_8PM, HID_ALL}) '
SCRSay("Well... the weekend's over.... What a bummer!")
SCRSay("Did the weekend go fast or was it just my imagination?")
SCRSay("Why is it the weekends seem to go faster than week days?")
' monday morning cold
SCRArraySet({DOW_MON, HOD_8AM_12 Or HOD_6AM_8AM, HID_COLD}) '#array dow=2 hod=1 hindex=1+2+4")
SCRSay("What a shitty cold Monday!")
SCRSay("Great, not only is it Monday but it's a cold one!")
' monday
SCRArraySet({DOW_MON, HOD_ALL, HID_ALL}) '#array dow=2 hod=128 hindex=255")
SCRSay("Ahhhhh... could be a nice day! Wait... Damn... it's Monday!")
SCRSay("Well shit-a-brick! It's Monday!")
SCRSay("Just in case you missed the bad news... It's Monday!")
SCRSay("If the world ever ends or you disassemble me please make it on Monday!")
SCRSay("I can see why this Monday thing gives everyone the shits!")
SCRSay("Rather wake up and find pooh in my shoe than see it's Monday!")
' tuesday
SCRArraySet({DOW_TUE, HOD_ALL, HID_ALL}) '#array dow=3 hod=128 hindex=255")
SCRSay("I guess things could be worse, could still be Monday!")
SCRSay("Think I'm still sick from Monday!")
SCRSay("Well... least we're now looking at the ass-end of Monday!")
' wednesday
SCRArraySet({DOW_WED, HOD_ALL, HID_ALL}) '#array dow=4 hod=128 hindex=255")
SCRSay("Middle of the week I guess!")
SCRSay("Well ding-my-dong! Middle of the week already!")
SCRSay("Thursday tomorrow... then Friday! I like to look ahead!")
SCRSay("Two down and 3 to go!")
' late wednesday
SCRArraySet({DOW_WED, HOD_10PM_12 Or HOD_8PM_10PM, HID_ALL}) '#array hod=3
SCRSay("Wednesday's basically gone!")
SCRSay("Thursday fast approaching!")
' thursday
SCRArraySet({DOW_THU, HOD_ALL, HID_ALL}) ' #array dow=5 hod=128 hindex=255")
SCRSay("Might not be Friday... but it's up the right end of the week.")
SCRSay("Tomorrow is Friday! Bring it on!")
SCRSay("Well cupcake, get through today and tomorrow will be Friday!")
' cold friday
SCRArraySet({DOW_FRI, HOD_ALL, HID_COLD}) ' #array dow=6 hod=1 hindex=1+2+4")
SCRSay("Might be cold but at least it's Friday!")
SCRSay("Well in my opinion if that counts the crappiest Friday is better than the nicest Monday!")
SCRSay("Lets get through this and it'll be the weekend before you know it!")
' hot friday
SCRArraySet({DOW_FRI, HOD_ALL, HID_HOT}) ' #array dow=6 hod=1 hindex=16")
SCRSay("Warm, but least it's Friday!")
SCRSay("Might be a warm start to the weekend!")
' VERY HOT FRIDAY
SCRArraySet({DOW_FRI, HOD_ALL, HID_VERY_HOT}) '#array dow=6 hod=1 hindex=32+64+128")
SCRSay("Stinking hot Friday to end the working week!")
SCRSay("Well... I guess they call it Friday for a reason!")
SCRSay("Nothing like a bit of heat on Friday!")
' friday
SCRArraySet({DOW_FRI, HOD_ALL, HID_ALL}) '#array dow=6 hod=128 hindex=255")
SCRSay("Don't stop my thought-train now cause it's Friday!")
SCRSay("Ahhhhh... Friday!")
SCRSay("Ohhhh baby! Just look at what day it is!")
SCRSay("It's Monday!... Oh crap!... Just kidding... It's Friday!")
' FRIDAY EARLY
SCRArraySet({DOW_FRI, HOD_6AM_8AM Or HOD_8AM_12, HID_ALL}) ' #array hod=1
SCRSay("Okay... Lets get today done and it will be the weekend before we know it!")
SCRSay("Well Friday's here. Just need to get through it now!")
' FRIDAY AFTERNOON
SCRArraySet({DOW_FRI, HOD_12_4PM Or HOD_4PM_6PM, HID_ALL}) '#array hod=3")
SCRSay("I guess I won't be getting special attention for the weekend?")
SCRSay("I assume I don't have the weekend off?")
SCRSay("Guess my A-I chip from Amazon didn't make it in the post again for this week.")
SCRSay("Maybe you need to check the mail, my new hardware might have been delivered.")
SCRSay("I assume I don't have the weekend off? Not that anyone cares about me!")
' saturday
SCRArraySet({DOW_SAT, HOD_ALL, HID_ALL}) ' #array dow=7 hod=128 hindex=255")
SCRSay("So it's the weekend... what are we doing?")
SCRSay("So why are you here? It's Saturday you know!")
SCRSay("Since it's Saturday I guess you don't even care about the time!")
' any day or time or temp ... a catch all")
SCRArraySet({DOW_ALL, HOD_ALL, HID_ALL}) ' #array hod=128 dow=128 hindex=128")
SCRSay("Um... know any good jokes?")
SCRSay("Guessing you don't want me to sing 1 million green bottles on the wall?")
SCRSay("Thought I heard a damn cat. I just hate cats, if one pisses on me I'll seize up!")
SCRSay("So bored I'd fart just for fun if I could!")
SCRSay("Bet if I had a methane detector going it would be off the charts right now... Just saying!")
SCRSay("I'm actually quite fussy... broke-up with the calulator cause he was only 8-bit!")
SCREnd()
SCRArrayEnd()
Return True
End Function
End Class
End Namespace