Hello All,
I am trying to set up a communication between 2 Teensy 4.1 devices using the Serial1 port (RX1, TX1 on pins 0 and 1). I set up a Master Teensy device that sends data and a Slave Teensy that receives data. Master Teensy runs a counter using a timer, and then sends the counter value to the Slave Teensy through Serial1. Both Teensy devices send the counter value (generated or received) through the USB Serial port.
My bug: the Slave device reports incorrect value every multiple of 256 counted value. It looks like the MSB of that value is loaded from the previous Serial1 transmission.
Any help or suggestion appreciated.
Code and capture of values received through USB are following:
Master Teensy below:
Slave Teensy below:
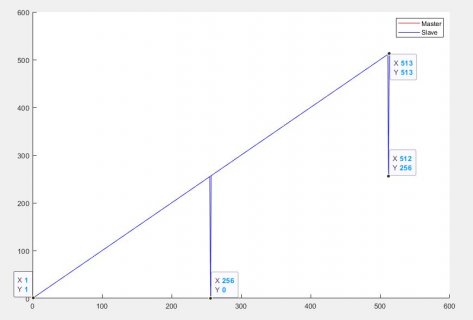
I am trying to set up a communication between 2 Teensy 4.1 devices using the Serial1 port (RX1, TX1 on pins 0 and 1). I set up a Master Teensy device that sends data and a Slave Teensy that receives data. Master Teensy runs a counter using a timer, and then sends the counter value to the Slave Teensy through Serial1. Both Teensy devices send the counter value (generated or received) through the USB Serial port.
My bug: the Slave device reports incorrect value every multiple of 256 counted value. It looks like the MSB of that value is loaded from the previous Serial1 transmission.
Any help or suggestion appreciated.
Code and capture of values received through USB are following:
Master Teensy below:
Code:
/*Master Teensy*/
#define RXTX_BAUD 4000000
IntervalTimer TXRX; // declares interval timer to impose a known period on USB received data
uint16_t kk_serial = 0;
void setup() {
if (RXTX_BAUD >= 6000000)
CCM_CSCDR1=105450240; // *0x0649_0B00 //UART_CLK_SEL bit set to 0 running from the 480MHz PLL
Serial1.begin(RXTX_BAUD);
TXRX.priority(1); // just reset is higher
TXRX.begin(TimerCall, 40); //TimerCall function runs every Sample_uS
}//end setup
void loop() {}// everything is done timed by an interval timer set in the setup()
void TimerCall()
{
if (kk_serial < 65535) // 2^16 count
{kk_serial = kk_serial+1;}
else
{kk_serial=0;}
Serial.write((char *)&kk_serial, 2); // same as above
Serial1.write((char *)&kk_serial, 2); // same as above
}
Slave Teensy below:
Code:
/*Slave Teensy*/
#define RXTX_BAUD 4000000
uint16_t kk_recvd = 0;
void setup() {
if (RXTX_BAUD >= 6000000)
CCM_CSCDR1=105450240; // *0x0649_0B00 UART_CLK_SEL bit set to 0 running from the 480MHz PLL
Serial1.begin(RXTX_BAUD);
}// setup() end
void loop() {
if(Serial1.available() > 1)
{ // last received read first: MSB, LSB
kk_recvd = ((Serial1.read()<<8) + Serial1.read());
TimeCall(); // calls timer function, but not a real timer
}
}// loop
void TimeCall()
{
Serial.write((char *)&kk_recvd, 2); // sends sample counter value as a 16 bit number to USB
Serial1.flush();
}
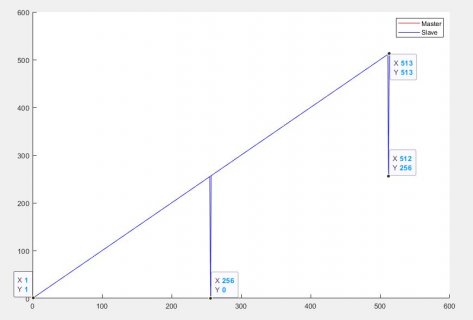