nicksalloum
Member
Hi there,
I'm currently experiencing some major lag on my LED setup. My current project specifications:
I have 4 LED strips, each 300 pixels long. Each strip is going to a separate pin on the Teensy.
With just 3 strips (Art-Net universes 0-1 to 5-140), the LEDs work really smoothly. The data comes through from Mad Mapper, and the effects show nicely. All 3 strips operate mostly fine, although very fast effects seem to drop some data. However, when I add the 4th strip (Art-Net universes 0-1 to 7-16), everything comes to a grinding halt. I'm a bit stuck on this one, and can't find much insights around after extensive searching. I'm assuming that I should be able to drive a lot more pixels over Art-Net based on the notes in the OctoWS2811 library.
Further notes:
In my circuitry, each data pin feeds into an input on the 74AHCT125 level shifter, and the output feeds into the strip. It's properly grounded and powered, and tested without Art-Net (using FastLED) just for safety.
I'm using the sample code from the OctoWS2811 library… the only difference is that I swapped out Ethernet and EthernetUdp for NativeEthernet and NativeEthernetUdp.
And in Mad Mapper, here's my DMX settings:
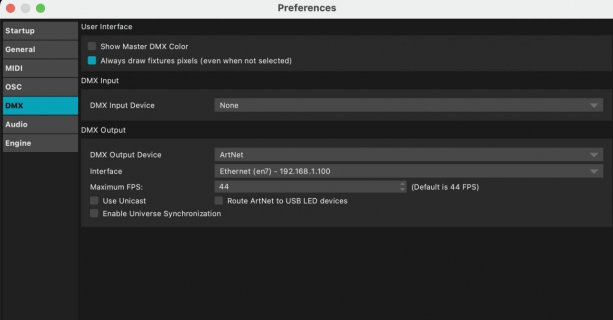
Any help would be greatly appreciated.
Thank you!
I'm currently experiencing some major lag on my LED setup. My current project specifications:
- Teensy 4.1
- Ethernet kit for Teensy 4.1 (https://www.pjrc.com/store/ethernet_kit.html)
- OctoWS2811 LED Library (https://www.pjrc.com/teensy/td_libs_OctoWS2811.html)
- Art-Net Library (https://github.com/natcl/Artnet)
- Mad Mapper for pixel mapping
I have 4 LED strips, each 300 pixels long. Each strip is going to a separate pin on the Teensy.
With just 3 strips (Art-Net universes 0-1 to 5-140), the LEDs work really smoothly. The data comes through from Mad Mapper, and the effects show nicely. All 3 strips operate mostly fine, although very fast effects seem to drop some data. However, when I add the 4th strip (Art-Net universes 0-1 to 7-16), everything comes to a grinding halt. I'm a bit stuck on this one, and can't find much insights around after extensive searching. I'm assuming that I should be able to drive a lot more pixels over Art-Net based on the notes in the OctoWS2811 library.
Further notes:
In my circuitry, each data pin feeds into an input on the 74AHCT125 level shifter, and the output feeds into the strip. It's properly grounded and powered, and tested without Art-Net (using FastLED) just for safety.
I'm using the sample code from the OctoWS2811 library… the only difference is that I swapped out Ethernet and EthernetUdp for NativeEthernet and NativeEthernetUdp.
Code:
// Receive multiple universes via Artnet and control a strip of ws2811 leds via OctoWS2811
//
// This example may be copied under the terms of the MIT license, see the LICENSE file for details
// https://github.com/natcl/Artnet
//
// http://forum.pjrc.com/threads/24688-Artnet-to-OctoWS2811?p=55589&viewfull=1#post55589
#include <Artnet.h>
#include <NativeEthernet.h>
#include <NativeEthernetUdp.h>
#include <SPI.h>
#include <OctoWS2811.h>
// Ideas for improving performance with WIZ820io / WIZ850io Ethernet:
// https://forum.pjrc.com/threads/45760-E1-31-sACN-Ethernet-DMX-Performance-help-6-Universe-Limit-improvements
// OctoWS2811 settings
const int numPins = 4;
byte pinList[numPins] = {9, 10, 11, 12};
const int ledsPerStrip = 300; // change for your setup
const byte numStrips= 4; // change for your setup
DMAMEM int displayMemory[ledsPerStrip*6];
int drawingMemory[ledsPerStrip*6];
const int config = WS2811_GRB | WS2811_800kHz;
OctoWS2811 leds(ledsPerStrip, displayMemory, drawingMemory, config, numPins, pinList);
// Artnet settings
Artnet artnet;
const int startUniverse = 0; // CHANGE FOR YOUR SETUP most software this is 1, some software send out artnet first universe as zero.
const int numberOfChannels = ledsPerStrip * numStrips * 3; // Total number of channels you want to receive (1 led = 3 channels)
byte channelBuffer[numberOfChannels]; // Combined universes into a single array
// Check if we got all universes
const int maxUniverses = numberOfChannels / 512 + ((numberOfChannels % 512) ? 1 : 0);
bool universesReceived[maxUniverses];
bool sendFrame = 1;
// Change ip and mac address for your setup
byte ip[] = {192, 168, 1, 10};
// byte broadcast[] = {192, 168, 1, 255};
byte mac[] = {0x04, 0xE9, 0xE5, 0x00, 0x69, 0xEC};
void setup()
{
Serial.begin(115200);
while (!Serial && millis() < 4000) {
// Wait for Serial
}
// artnet.setBroadcast(broadcast);
artnet.begin(mac, ip);
leds.begin();
initTest();
// this will be called for each packet received
artnet.setArtDmxCallback(onDmxFrame);
}
void loop()
{
// we call the read function inside the loop
artnet.read();
}
void onDmxFrame(uint16_t universe, uint16_t length, uint8_t sequence, uint8_t* data)
{
sendFrame = 1;
// Store which universe has got in
if (universe < maxUniverses)
universesReceived[universe] = 1;
for (int i = 0 ; i < maxUniverses ; i++)
{
if (universesReceived[i] == 0)
{
// Serial.println("Broke");
sendFrame = 0;
break;
}
}
// read universe and put into the right part of the display buffer
for (int i = 0 ; i < length ; i++)
{
int bufferIndex = i + ((universe - startUniverse) * length);
if (bufferIndex < numberOfChannels) // to verify
channelBuffer[bufferIndex] = byte(data[i]);
}
// send to leds
for (int i = 0; i < ledsPerStrip * numStrips; i++)
{
leds.setPixel(i, channelBuffer[(i) * 3], channelBuffer[(i * 3) + 1], channelBuffer[(i * 3) + 2]);
}
if (sendFrame)
{
leds.show();
// Reset universeReceived to 0
memset(universesReceived, 0, maxUniverses);
}
}
void initTest()
{
for (int i = 0 ; i < ledsPerStrip * numStrips ; i++)
leds.setPixel(i, 127, 0, 0);
leds.show();
delay(500);
for (int i = 0 ; i < ledsPerStrip * numStrips ; i++)
leds.setPixel(i, 0, 127, 0);
leds.show();
delay(500);
for (int i = 0 ; i < ledsPerStrip * numStrips ; i++)
leds.setPixel(i, 0, 0, 127);
leds.show();
delay(500);
for (int i = 0 ; i < ledsPerStrip * numStrips ; i++)
leds.setPixel(i, 0, 0, 0);
leds.show();
}
And in Mad Mapper, here's my DMX settings:
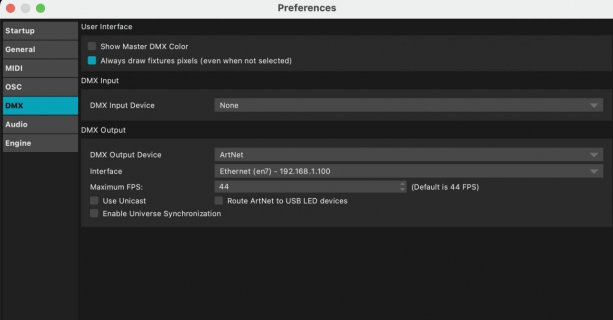
Any help would be greatly appreciated.
Thank you!