carlsuttle
Member
I really struggled to find and get addr2line to work. Here is how I did that.
In the IDE set File/Preferences compile and upload tick boxes
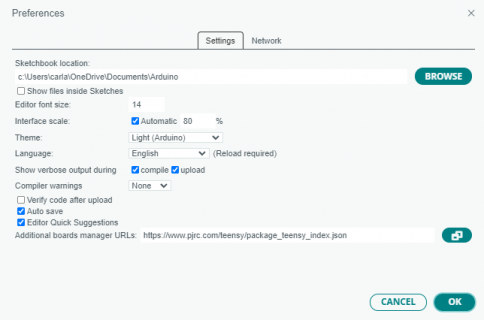
Add an example line of code to your sketch from https://github.com/PaulStoffregen/MyFault/tree/main/examples
I used Data Access Violation and added the lines below to my code.
Compile and upload the sketch. The IDE reports:
From which we note the path of the
Now force the error with the serial monitor open. After o.5 seconds the error was forced (presumably, and 8 seconds later the Teensy rebooted.
In the serial monitor after reboot I saw:
Now to find the sketch line number.
Make a note of the address from the crash report "0x93A"
Navigate to: the tools folder, Tools folder "C:\Users\carla\AppData\Local\Arduino15\packages\teensy\tools"
and navigate further to "C:\Users\carla\AppData\Local\Arduino15\packages\teensy\tools\teensy-compile\5.4.1\arm\bin" where you should find "arm-none-eabi-addr2line.exe"
I used notepad to construct the following text so I can paste it into the Command window, its a lot easier.
"arm-none-eabi-addr2line -e C:/Users/carla/AppData/Local/Temp/arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf 0x93A"
Which returns in the Command Window:
"C:\Users\carla\OneDrive - n-ats.com\Engineering\HID Project\Yoke_Stick_v2.5_sno_ny/Yoke_Stick_v2.5_sno_ny.ino:493"
Looking up line 493 in the sketch gives us the line which caused the error:
"*(volatile uint32_t *)0x30000000 = 0; // causes Data_Access_Violation"
That's it, phew. Now maybe I can find out if my teensy crashes just where and when it did it.
P.S. Note I also added this line from the example:
And the crash report included "Breadcrumb #1 was 83886572 (0x50001EC)"
01EC is 492 decimal. The line of the sketch when the breadcrumb was written to.
That's useful:
In the IDE set File/Preferences compile and upload tick boxes
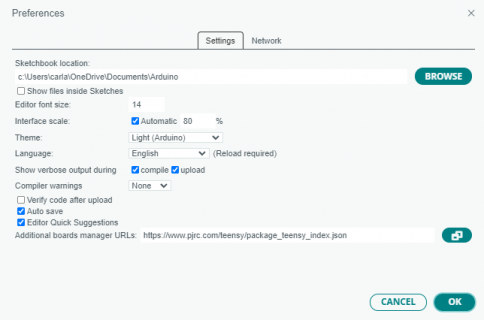
Add an example line of code to your sketch from https://github.com/PaulStoffregen/MyFault/tree/main/examples
I used Data Access Violation and added the lines below to my code.
Code:
void setup() {
if ( CrashReport ) {
while (!Serial && millis() < 10000 );
Serial.println("\n" __FILE__ " " __DATE__ " " __TIME__);
Serial.print(CrashReport);
}
.........
....somewhere in the main loop....
if (outputlog == 4) {
sprintf(buff, "Data access violation forced in 0.5 second");
DEBUG_PRINTLN(buff);
delay(500);
CrashReport.breadcrumb( 1, 0x5000000 | __LINE__ ); // Upper bits hold '5' perhaps indicating func() for ref, lower bits show line #
*(volatile uint32_t *)0x30000000 = 0; // causes Data_Access_Violation
}
Compile and upload the sketch. The IDE reports:
Code:
Linking everything together...
"C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-compile\\5.4.1/arm/bin/arm-none-eabi-gcc" -O2 -Wl,--gc-sections,--relax "-TC:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\hardware\\avr\\1.57.2\\cores\\teensy4/imxrt1062.ld" -mthumb -mcpu=cortex-m7 -mfloat-abi=hard -mfpu=fpv5-d16 -o "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702\\sketch\\Yoke_Stick_v2.5_sno_ny.ino.cpp.o" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702\\libraries\\PWMServo\\PWMServo.cpp.o" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702\\libraries\\SimpleKalmanFilter\\SimpleKalmanFilter.cpp.o" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702\\libraries\\HX711\\HX711.cpp.o" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702\\libraries\\EEPROM\\EEPROM.cpp.o" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/..\\arduino-core-cache\\core_8c54a49357957ff2477bf8e4c39c2077.a" "-LC:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702" -larm_cortexM7lfsp_math -lm -lstdc++
"C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-compile\\5.4.1/arm/bin/arm-none-eabi-objcopy" -O ihex -j .eeprom --set-section-flags=.eeprom=alloc,load --no-change-warnings --change-section-lma .eeprom=0 "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.eep"
"C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-compile\\5.4.1/arm/bin/arm-none-eabi-objcopy" -O ihex -R .eeprom "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.hex"
"C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-tools\\1.57.2/teensy_secure" encrypthex TEENSY40 "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.hex"
No key .pem file found, skipping .ehex encryption
"C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-tools\\1.57.2/teensy_post_compile" -file=Yoke_Stick_v2.5_sno_ny.ino "-path=C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702" "-tools=C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-tools\\1.57.2/" -board=TEENSY40
"C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-tools\\1.57.2/stdout_redirect" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.sym" "C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-compile\\5.4.1/arm/bin/arm-none-eabi-objdump" -t -C "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf"
Memory Usage on Teensy 4.0:
FLASH: code:38240, data:10216, headers:8884 free for files:1974276
RAM1: variables:12512, code:34728, padding:30808 free for local variables:446240
RAM2: variables:2176 free for malloc/new:522112
"C:\\Users\\carla\\AppData\\Local\\Arduino15\\packages\\teensy\\tools\\teensy-tools\\1.57.2/teensy_size" "C:\\Users\\carla\\AppData\\Local\\Temp\\arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf"
From which we note the path of the
- Tools folder "C:\Users\carla\AppData\Local\Arduino15\packages\teensy\tools"
- And .elf file "C:/Users/carla/AppData/Local/Temp/arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf"
Now force the error with the serial monitor open. After o.5 seconds the error was forced (presumably, and 8 seconds later the Teensy rebooted.
In the serial monitor after reboot I saw:
Code:
09:56:38.282 -> C:\Users\carla\OneDrive - n-ats.com\Engineering\HID Project\Yoke_Stick_v2.5_sno_ny\Yoke_Stick_v2.5_sno_ny.ino Jan 5 2023 09:21:31
09:56:38.282 -> CrashReport:
09:56:38.282 -> A problem occurred at (system time) 9:56:29
09:56:38.282 -> Code was executing from address 0x93A
09:56:38.282 -> CFSR: 82
09:56:38.282 -> (DACCVIOL) Data Access Violation
09:56:38.282 -> (MMARVALID) Accessed Address: 0x30000000
09:56:38.282 -> Temperature inside the chip was 60.34 °C
09:56:38.282 -> Startup CPU clock speed is 600MHz
09:56:38.282 -> Reboot was caused by auto reboot after fault or bad interrupt detected
09:56:38.282 -> Breadcrumb #1 was 83886572 (0x50001EC)
Now to find the sketch line number.
Make a note of the address from the crash report "0x93A"
Navigate to: the tools folder, Tools folder "C:\Users\carla\AppData\Local\Arduino15\packages\teensy\tools"
and navigate further to "C:\Users\carla\AppData\Local\Arduino15\packages\teensy\tools\teensy-compile\5.4.1\arm\bin" where you should find "arm-none-eabi-addr2line.exe"
I used notepad to construct the following text so I can paste it into the Command window, its a lot easier.
"arm-none-eabi-addr2line -e C:/Users/carla/AppData/Local/Temp/arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf 0x93A"
- Open a Command Prompt (Console) window.
- CD to "C:\Users\carla\AppData\Local\Arduino15\packages\teensy\tools\teensy-compile\5.4.1\arm\bin"
- Add the command (from notepad) "arm-none-eabi-addr2line -e C:/Users/carla/AppData/Local/Temp/arduino-sketch-8AF392A524185D95185266EB754B8702/Yoke_Stick_v2.5_sno_ny.ino.elf 0x93A"
Which returns in the Command Window:
"C:\Users\carla\OneDrive - n-ats.com\Engineering\HID Project\Yoke_Stick_v2.5_sno_ny/Yoke_Stick_v2.5_sno_ny.ino:493"
Looking up line 493 in the sketch gives us the line which caused the error:
"*(volatile uint32_t *)0x30000000 = 0; // causes Data_Access_Violation"
That's it, phew. Now maybe I can find out if my teensy crashes just where and when it did it.
P.S. Note I also added this line from the example:
Code:
CrashReport.breadcrumb( 1, 0x5000000 | __LINE__ ); // Upper bits hold '5' perhaps indicating func() for ref, lower bits show line #
01EC is 492 decimal. The line of the sketch when the breadcrumb was written to.
That's useful:
Last edited: