luni
Well-known member
webHID feasibility experiments
I did a few feasibility experiments with the webHID interface of chrome. This interface allows a web page to communicate with a Teensy over its HID interface. Since firmware uploading is also done over HID, the webHID interface would also allow firmware uploads through a web browser. If someone is interested, here some information and a example which shows how to exchange hid reports between a webpage and a Teensy. (I only tested the Chrome implementation of the interface. No idea if other browsers support it as well).
Here instructions for a quick start
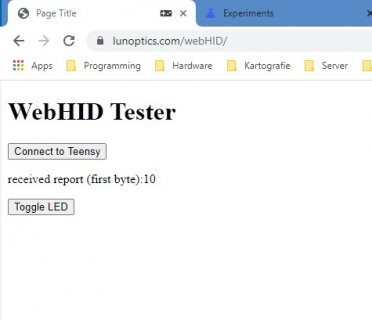
The connect button opens a browser generated list box which lists all raw hid Teensies. I.e. all boards with vid: 0x16C0, pid: 0x486, usagepage: 0xFFAB, usage: 0x200. Select the the one you want to connect to.
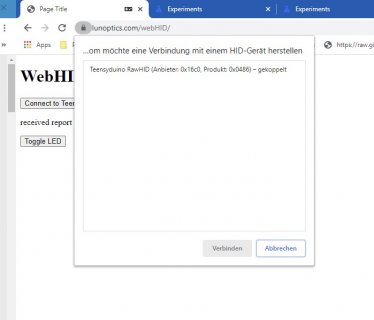
If everything works you should see the incrementing bytes as they are sent from the Teensy. You can toggle the LED on your board by pressing the Toggle button.
index.html
And here the corresponding java script. Please note that I and java script probably won't get friends in this life. So, this probably can be done much better and more elegant. For the time being I'm happy that it works at all
Github Repo: https://github.com/luni64/webHID
General info about webHID https://github.com/robatwilliams/awesome-webhid
I did a few feasibility experiments with the webHID interface of chrome. This interface allows a web page to communicate with a Teensy over its HID interface. Since firmware uploading is also done over HID, the webHID interface would also allow firmware uploads through a web browser. If someone is interested, here some information and a example which shows how to exchange hid reports between a webpage and a Teensy. (I only tested the Chrome implementation of the interface. No idea if other browsers support it as well).
Here instructions for a quick start
- To enable the Interface in Chrome browse to chrome://flags/ , search for "Experimental Web Platform features" and change the flag to "Enabled"
- Compile the following test firmware with usb option "RAW HID".
Code:#include "Arduino.h" void setup() { pinMode(LED_BUILTIN, OUTPUT); } uint8_t cnt = 0; uint8_t outgoingReport[64]; uint8_t incommingReport[64]; void loop() { // sending report outgoingReport[0] = cnt++; usb_rawhid_send(outgoingReport, 100); // receiving report if (usb_rawhid_recv(incommingReport, 100) > 0) // Wait for a received raw hid report { if (incommingReport[0] == 0xAA ) { digitalToggleFast(LED_BUILTIN); } } delay(100); }
- Browse to the test web page: https://lunoptics.com/webHID/ where you can clearly see that I'm not a web designer
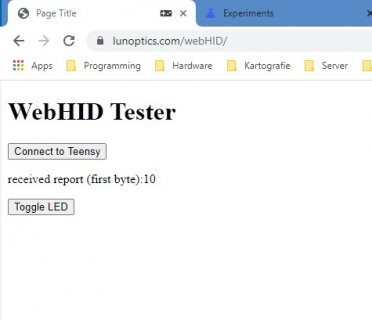
The connect button opens a browser generated list box which lists all raw hid Teensies. I.e. all boards with vid: 0x16C0, pid: 0x486, usagepage: 0xFFAB, usage: 0x200. Select the the one you want to connect to.
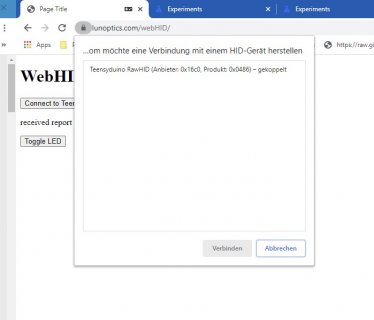
If everything works you should see the incrementing bytes as they are sent from the Teensy. You can toggle the LED on your board by pressing the Toggle button.
index.html
Code:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>WebHID Tester</h1>
<button type="button" id="connectButton">Connect to Teensy</button>
<p id="inputReport">no data</p>
<button type="button" id="sendButton">Toggle LED</button>
<script src="js/main.js"></script>
</body>
</html>
And here the corresponding java script. Please note that I and java script probably won't get friends in this life. So, this probably can be done much better and more elegant. For the time being I'm happy that it works at all
Code:
let requestButton = document.getElementById('connectButton');
let ledButton = document.getElementById('sendButton');
let inputReportDisplay = document.getElementById('inputReport')
let rawHidFilter = { vendorId: 0x16C0, productId: 0x0486, usagePage: 0xFFAB, usage: 0x0200 }; // RawHID interface
let serEmuFilter = { vendorId: 0x16C0, productId: 0x0486, usagePage: 0xFFC9, usage: 0x0004 }; // SerEMU interface
let rawHidParams = { filters: [rawHidFilter] };
let serEmuParams = { filters: [serEmuFilter] };
let outputReportId = 0x00;
let outputReport = new Uint8Array([64]);
let device;
function handleInputReport(e) {
let firstByte = e.data.getUint8(0); // read first byte of 64byte report
inputReportDisplay.innerHTML = "received report (first byte):" + firstByte;
};
function toggleLED()
{
outputReport[0] = 0xAA; // send a report with some data to the Teensy
device.sendReport(outputReportId,outputReport);
}
async function connect() {
let devices = await navigator.hid.requestDevice(rawHidParams); //get allowance to connect to a rawHID Teensy
if (devices === null || devices.length == 0) return;
device = devices[0];
console.log('found device: ' + device.productName);
if (device.open()) {
console.log('Opened device: ' + device.productName);
device.addEventListener('inputreport', handleInputReport);
};
};
requestButton.addEventListener('click', connect);
ledButton.addEventListener('click', toggleLED);
Github Repo: https://github.com/luni64/webHID
General info about webHID https://github.com/robatwilliams/awesome-webhid
Last edited by a moderator: