Hi,
This is related to the OctoWS2811 flicker when connected via USB thread, but after further testing, USB is not the root cause or even necessary to show the problem.
It seems that OctoWS2811 has bus arbitration issues with the DMA whenever the CPU is doing anything else. With a small strip of 100 WS2812B LEDs (from Adafruit here, with the OctoWS2811 adapter board), the following code flickers very badly, particularly towards the end of the strip:
Looking at it with a logic analyzer, I sampled the Teensy's output for 10 seconds at 50 MHz and plotted a histogram of the "1" pulse widths. Here are all pulses shorter than 400ns:
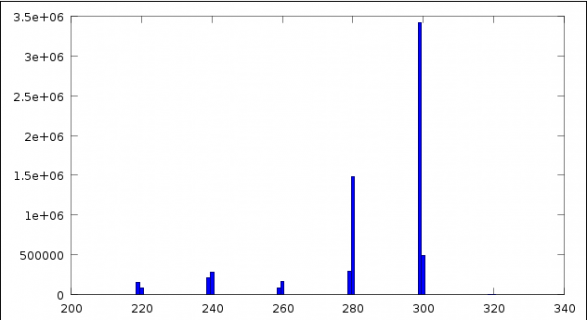
Here are the pulses longer than 400ns:
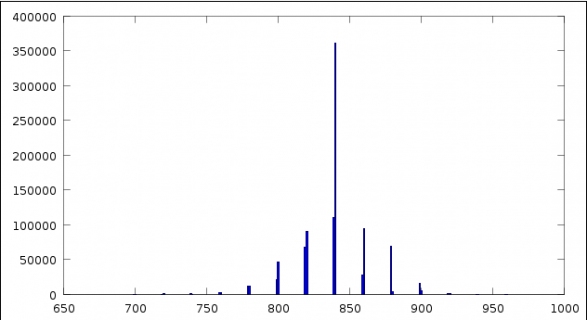
The short pulses around 220ns are probably causing at least some of the problem -- looking further down the chain of LEDs, it appears that they're too short, and getting dropped. I think this is happening because the DMA that sets the output high is getting delayed. There may be other problems too; this is just the most obvious.
The raw data for those histograms is:
This is related to the OctoWS2811 flicker when connected via USB thread, but after further testing, USB is not the root cause or even necessary to show the problem.
It seems that OctoWS2811 has bus arbitration issues with the DMA whenever the CPU is doing anything else. With a small strip of 100 WS2812B LEDs (from Adafruit here, with the OctoWS2811 adapter board), the following code flickers very badly, particularly towards the end of the strip:
Code:
#include <OctoWS2811.h>
#define STRIP_LEN 100
static DMAMEM int displayMem[STRIP_LEN * 8];
static int drawMem[STRIP_LEN * 8];
OctoWS2811 octo(STRIP_LEN, displayMem, drawMem, WS2811_GRB | WS2811_800kHz);
void setup()
{
Serial.begin(115200);
octo.begin();
for (int i = 0; i < STRIP_LEN * 8; i++)
octo.setPixel(i, 0x010101);
}
void loop()
{
static float foo = 1234.45;
octo.show();
while (octo.busy()) {
float m = cos(sin(millis())) + 10;
foo *= m;
foo /= m;
}
}
Looking at it with a logic analyzer, I sampled the Teensy's output for 10 seconds at 50 MHz and plotted a histogram of the "1" pulse widths. Here are all pulses shorter than 400ns:
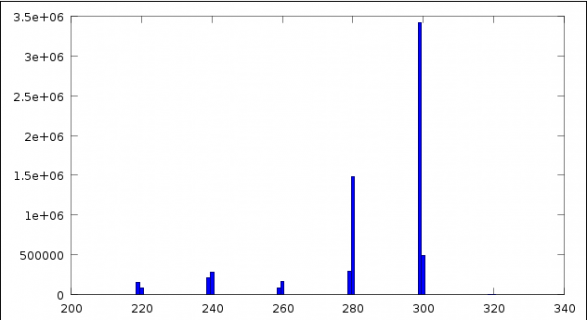
Here are the pulses longer than 400ns:
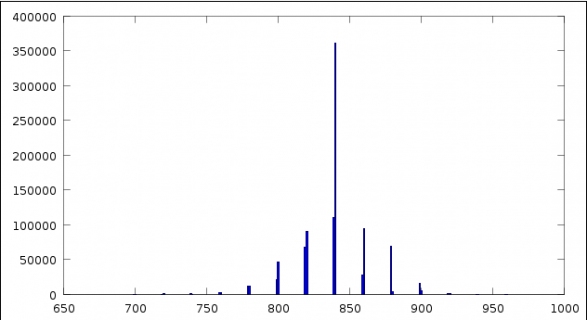
The short pulses around 220ns are probably causing at least some of the problem -- looking further down the chain of LEDs, it appears that they're too short, and getting dropped. I think this is happening because the DMA that sets the output high is getting delayed. There may be other problems too; this is just the most obvious.
The raw data for those histograms is:
Code:
219 146003
220 86207
239 215491
240 274804
259 86392
260 166190
279 294279
280 1484490
299 3423085
300 487988
319 3080
320 1179
699 148
700 164
719 373
720 1142
739 1923
740 506
759 2865
760 2059
779 11777
780 11783
799 21832
800 46214
819 68660
820 90952
839 110116
840 361677
859 28411
860 94094
879 68680
880 4320
899 16555
900 5197
919 1336
920 681
939 524
940 631
959 80
960 41