Hello, I'm encountering a strange problems I haven't seen before on Teensy (using Teensy 4.1). I am writing a test platform that uses SPI and am encountering an issue where the CS returns high before the SPI. transfer completes. You can see this in the attached oscilloscope image on the yellow waveform. I am using PlatformIO as the programming environment and the Teensy is running at default settings (600 MHz, etc.). I have tried running this code from via PlatformIO on both Windows and MAC and see the same result. So far I have tried switching CS pins, digitalWrite and digitalWriteFast, creating a new project from scratch, and changing the SPI frequency. The only solution I have encountered so far is to place a delay (commented out in the code below) to block the CS from going high until the transactions have completed. I wanted to attempt using the Arduino IDE but I am having trouble getting 2.X running on my laptop due to some javascript issues.
I have been using Teensys for about 7 years now and have never seen anything like this, but it has been about a year since I have done any development with this platform so its possible I am making a dumb mistake but what I am doing now looks pretty much identical to my code for previous chips which functions. Any ideas or assistance would be greatly appreciated!
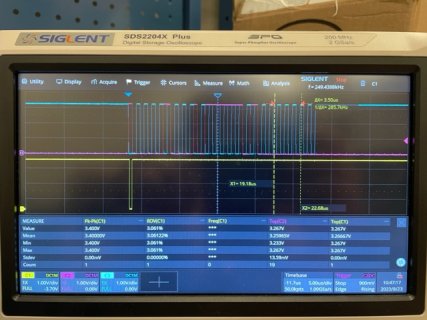
I have been using Teensys for about 7 years now and have never seen anything like this, but it has been about a year since I have done any development with this platform so its possible I am making a dumb mistake but what I am doing now looks pretty much identical to my code for previous chips which functions. Any ideas or assistance would be greatly appreciated!
Code:
// Include necessary Arduino libraries
#include <Arduino.h>
#include <SPI.h>
// Include local libraries and headers
#include "pin_definitions.h"
void setup()
{
SPI.begin();
pinMode(SPI_CS_DAC_PIN, OUTPUT);
digitalWrite(SPI_CS_DAC_PIN, HIGH);
}
void loop()
{
SPI.beginTransaction(SPISettings(1000000, MSBFIRST, SPI_MODE0));
digitalWrite(SPI_CS_DAC_PIN, LOW);
SPI.transfer(0x08);
SPI.transfer16(32000);
// delayMicroseconds(29);
digitalWrite(SPI_CS_DAC_PIN, HIGH);
SPI.endTransaction();
delay(1000);
}
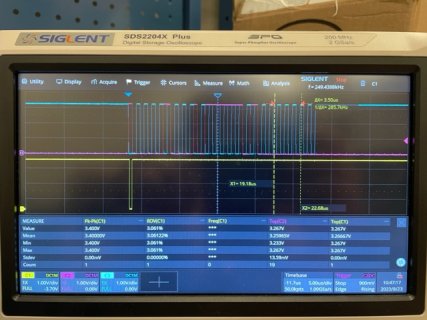